- [ between the brackets ]
- Posts
- Go vs Rust vs C: An in-depth Analysis
Go vs Rust vs C: An in-depth Analysis
The Fast and the Furious
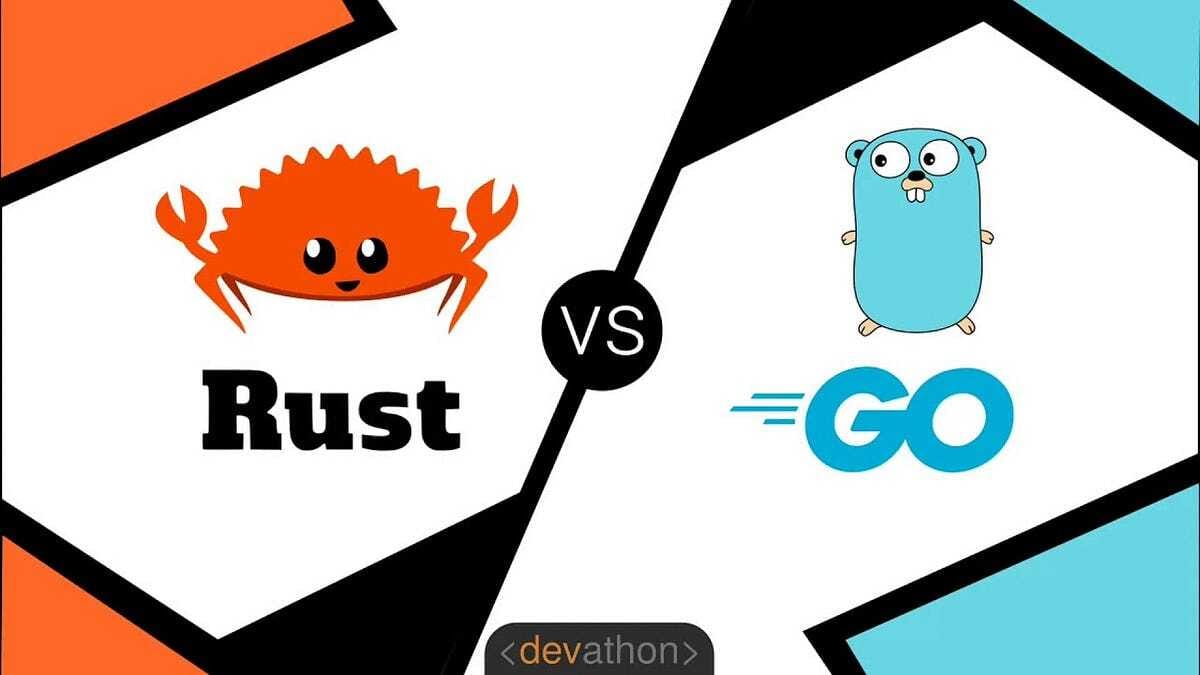
The Old but Gold: C
C is a long-standing, highly influential language that helped shape many others we use today. It's often praised for its efficiency and direct control over hardware. You'll see it frequently in system programming, game development, and other performance-critical applications.
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
C Pros:
Efficient and fast execution
Direct access to low-level hardware
Broad support in compilers, libraries, and operating systems
C Cons:
Manual memory management can lead to bugs and security issues
Less safe due to the lack of modern programming features
Steeper learning curve compared to some modern languages
C's power is its precision and control, but these come with complexity. Let's consider the Heartbleed bug. This infamous OpenSSL vulnerability was due to a memory handling mistake — an error that's easy to make in C, with its manual memory management.
The Simplistic Stalwart: Go
Go, developed by Google, champions simplicity and efficiency. It's often used for network servers, data pipelines, and other back-end systems due to its simplicity and in-built concurrency support.
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Go Pros:
Simple syntax and semantics make it easy to learn and read
Built-in concurrency model (goroutines and channels)
Automatic memory management and garbage collection
Go Cons:
Lack of generics can make code duplication necessary
Smaller standard library compared to some languages
Error handling can be verbose
Go shines in Docker, the popular containerization platform. Docker's server and CLI client are written in Go, illustrating how Go's simplicity and performance work hand-in-hand for network-heavy, concurrent tasks.
The Safe and Speedy: Rust
Rust, a product of Mozilla, is known for its focus on safety and performance. Rust's primary selling point is that it provides memory safety without a garbage collector. Rust is frequently used in system programming, game development, and web assembly.
fn main() {
println!("Hello, World!");
}
Rust Pros:
High performance, comparable to C and C++
Strong static typing and ownership model prevent many types of bugs
Modern language features like pattern matching and type inference
Rust Cons:
Steeper learning curve due to unique concepts like ownership and borrowing
Longer compilation times
Smaller ecosystem compared to more established languages
Firecracker, a virtualization technology from AWS, is written in Rust. It demonstrates Rust's utility in high-performance, safety-critical applications where a single memory mistake could have devastating consequences.
Performance
When it comes to performance, the host language plays an important role but it doesn't tell the whole story. This becomes especially apparent when we take a look at the results from The Computer Language Benchmarks Game, which compares how these languages perform across different tasks.
Fannkuch-Redux
The Fannkuch-Redux test measures the performance of raw CPU power and cache effects. The fastest Rust program completes this task in 3.51 seconds, using about 11,036 kilobytes of memory and taking up 13.93 CPU seconds. Meanwhile, the fastest Go program takes 8.25 seconds, uses 10,936 kilobytes of memory, and utilizes 32.92 CPU seconds.
Interestingly, even the fastest C program takes 2.05 seconds, almost half of Rust's time, and 11,236 kilobytes of memory, proving that when it comes to raw computational power, C is tough to beat.
N-Body
The N-Body simulation measures the performance of raw CPU power in a different context: floating-point math. Rust does well here too, with its fastest program taking just 2.81 seconds. Go lags behind with a time of 6.36 seconds, and C leading the pack with a record time of 2.21 seconds.
Spectral-Norm
The spectral-norm benchmark is yet another test that puts raw CPU power to the test. The fastest Rust program completes the task in 0.71 seconds, using about 11,124 kilobytes of memory and taking up 2.84 CPU seconds. Go, on the other hand, took much longer at 5.31 seconds with the same memory usage and more CPU seconds at 5.32. Again, C shows its performance prowess with a speedy 0.39 seconds.
Mandelbrot
The Mandelbrot test is a measure of both CPU performance and memory management. The best Rust program completes in 1.01 seconds, with Go trailing behind at 3.73 seconds. Once again, C comes out ahead with the top performing program clocking in at just 0.52 seconds.
Binary-Trees
The binary-trees benchmark tests memory allocation and deallocation speed. Rust completes this task at 1.08 seconds, while Go takes significantly longer with a time of 27.82 seconds, pointing towards more efficient memory management in Rust. But C shines again with a completion time of just 0.80 seconds.
Comparative Analysis
When compared, each language has its unique strengths and weaknesses. C offers unparalleled control and speed but sacrifices safety. Go provides simplicity and efficient concurrency handling but lacks some common modern features. Rust delivers safety and performance but requires learning new paradigms.
Your choice depends on the specific use case, the project's complexity, the need for speed, safety, or simplicity.
[ Zach Coriarty ]